How to Get a LINE User MID
When getting started with LINE API messaging, you need to know the mid
of a message recipient. It’s not his/her username. It’s a string that looks like ub8dbd4a12c322f6c0118883d839c55a4
.
LINE utilizes a callback URL that you can set for your trial LINE bot. At this endpoint you can place a script, shown below, which will report your mid
back to you when you type “whoami”.
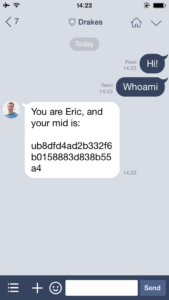
You need the LINE PHP SDK installed for this script below. I recommend installing it with composer.
After you have installed the SDK and have created your trial LINE bot account, register a callback URL as per the API instructions (e.g. https://example.com:443/callback/
).
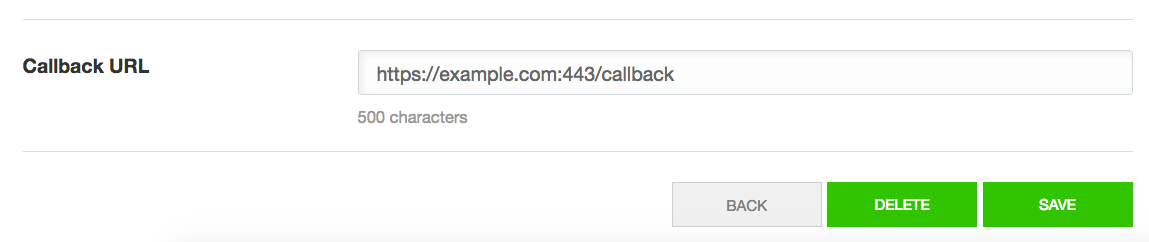
After that, using whichever device has the account of the mid
you wish to know, scan the QR code in the Channel Console and add your trial LINE bot to your LINE account. After you have added your trial LINE bot as your friend, send “whoami” to it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 | <?php /** * Eric Draken * Date: 2016-09-02 * Time: 4:44 PM * Desc: Callback for responding to Line messages * Send 'whoami' to this endpoint to get a reply with your mid. */ // I put constants like 'LINE_CHANNEL_ID' here //require_once __DIR__ . '/../includes/config.php'; require_once __DIR__ . "/../includes/line-bot-sdk/vendor/autoload.php"; use LINE\LINEBot; use LINE\LINEBot\HTTPClient\GuzzleHTTPClient; // Set these values $config = [ 'channelId' => LINE_CHANNEL_ID, 'channelSecret' => LINE_CHANNEL_SECRET, 'channelMid' => LINE_CHANNEL_MID, ]; $sdk = new LINEBot($config, new GuzzleHTTPClient($config)); $postdata = @file_get_contents("php://input"); $messages = $sdk->createReceivesFromJSON($postdata); // Verify the signature // REF: http://line.github.io/line-bot-api-doc/en/api/callback/post.html#signature-verification // REF: http://stackoverflow.com/a/541450 $sigheader = 'X-LINE-ChannelSignature'; $signature = @$_SERVER[ 'HTTP_'.strtoupper(str_replace('-','_',$sigheader)) ]; if($signature && $sdk->validateSignature($postdata, $signature)) { // Next, extract the messages if(is_array($messages)) { foreach ($messages as $message) { if ($message instanceof LINEBot\Receive\Message\Text) { $text = $message->getText(); if (strtolower(trim($text)) === "whoami") { $fromMid = $message->getFromMid(); $user = $sdk->getUserProfile($fromMid); $displayName = $user['contacts'][0]['displayName']; $reply = "You are $displayName, and your mid is:\n\n$fromMid"; // Send the mid back to the sender and check if the message was delivered $result = $sdk->sendText([$fromMid], $reply); if(!$result instanceof LINE\LINEBot\Response\SucceededResponse) { error_log('LINE error: ' . json_encode($result)); } } else { // Process normally, or do nothing } } else { // Process other types of LINE messages like image, video, sticker, etc. } } } // Else, error } else { error_log('LINE signatures didn\'t match: ' . $signature); } |
This above script is a simple bootstrap to make a more functional bot, and it incorporates the recommended signature verification.